FlowRunner Component
The CustomComponent class allows us to create components that interact with Langflow itself. In this example, we will make a component that runs other flows available in "My Collection".
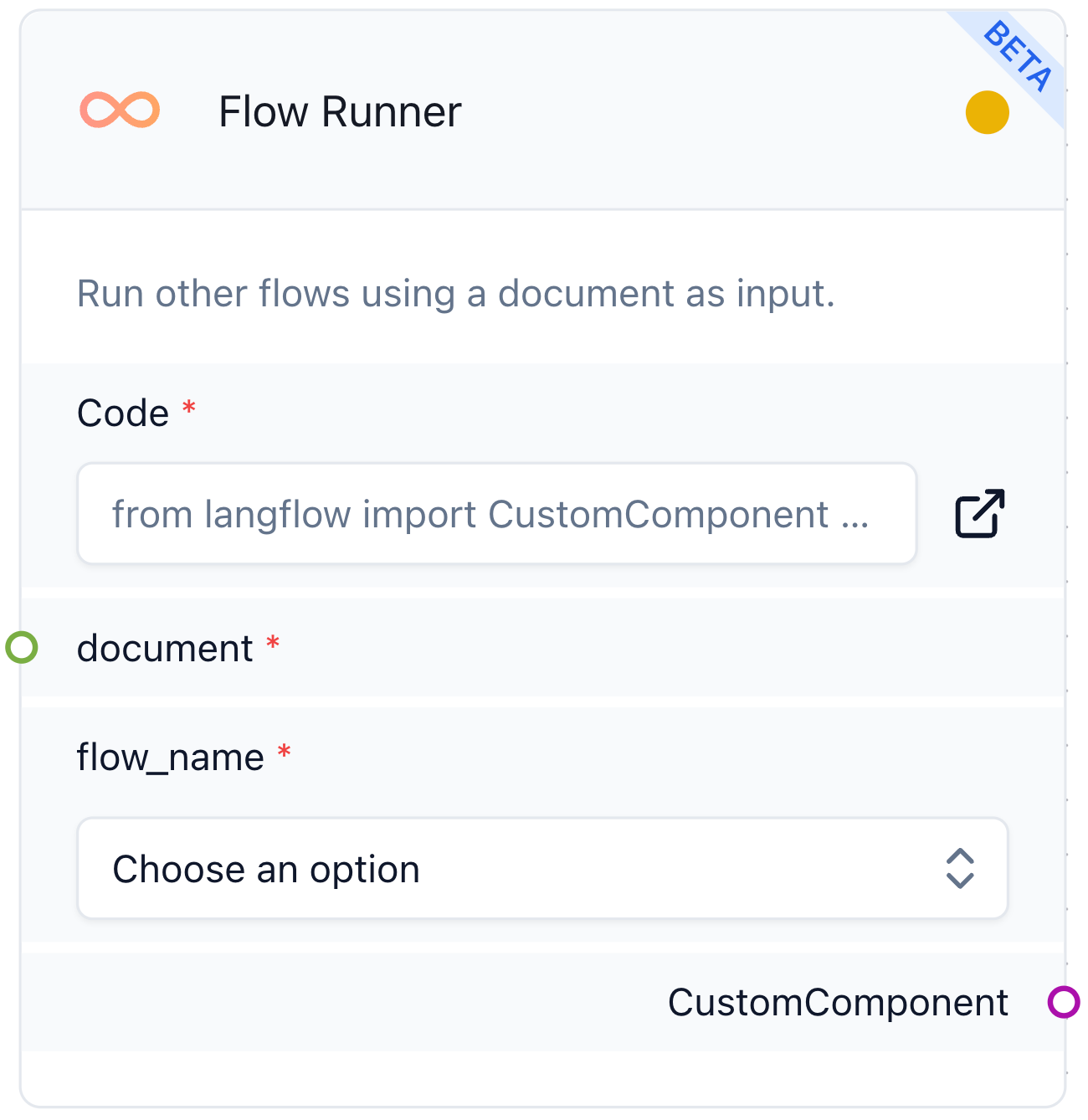
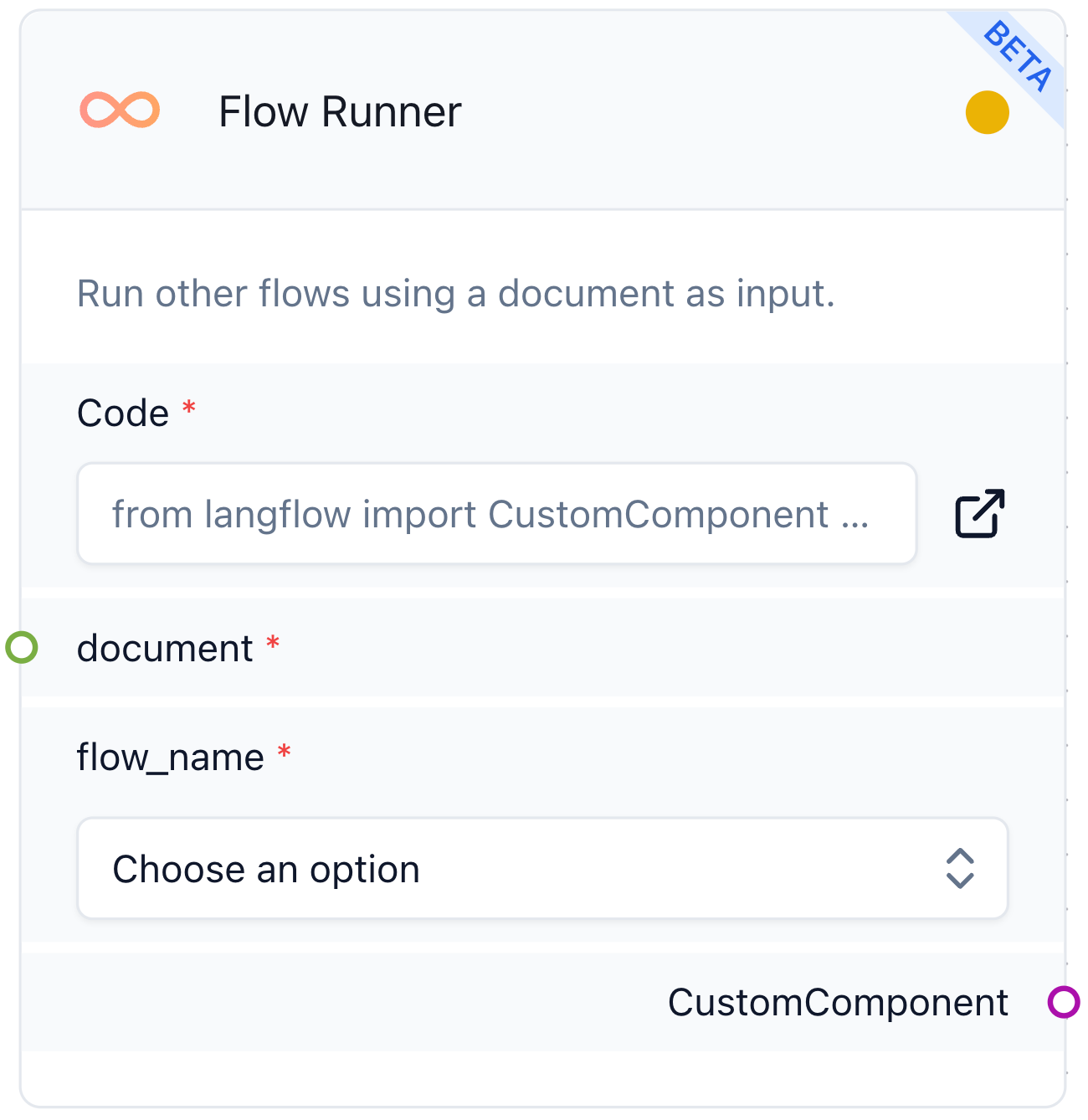
We will cover how to:
- List Collection flows using the
list_flows
method. - Load a flow using the
load_flow
method. - Configure a dropdown input field using the
options
parameter.
Example Code
_32from langflow import CustomComponent_32from langchain.schema import Document_32_32class FlowRunner(CustomComponent):_32 display_name = "Flow Runner"_32 description = "Run other flows using a document as input."_32_32 def build_config(self):_32 flows = self.list_flows()_32 flow_names = [f.name for f in flows]_32 return {"flow_name": {"options": flow_names,_32 "display_name": "Flow Name",_32 },_32 "document": {"display_name": "Document"}_32 }_32_32_32 def build(self, flow_name: str, document: Document) -> Document:_32 # List the flows_32 flows = self.list_flows()_32 # Get the flow that matches the selected name_32 # You can also get the flow by id_32 # using self.get_flow(flow_id=flow_id)_32 tweaks = {}_32 flow = self.get_flow(flow_name=flow_name, tweaks=tweaks)_32 # Get the page_content from the document_32 if document and isinstance(document, list):_32 document = document[0]_32 page_content = document.page_content_32 # Use it in the flow_32 result = flow(page_content)_32 return Document(page_content=str(result))
_12from langflow import CustomComponent_12_12_12class MyComponent(CustomComponent):_12 display_name = "Custom Component"_12 description = "This is a custom component"_12_12 def build_config(self):_12 ..._12_12 def build(self):_12 ..._12_12_12_12_12_12_12_12
The typical structure of a Custom Component is composed of display_name
and description
attributes, build
and build_config
methods.
_12from langflow import CustomComponent_12_12_12class FlowRunner(CustomComponent):_12 display_name = "Flow Runner"_12 description = "Run other flows"_12_12 def build_config(self):_12 ..._12_12 def build(self):_12 ..._12_12_12_12_12_12_12_12
Let's start by defining our component's display_name
and description
.
_13from langflow import CustomComponent_13from langchain.schema import Document_13_13_13class FlowRunner(CustomComponent):_13 display_name = "Flow Runner"_13 description = "Run other flows using a document as input."_13_13 def build_config(self):_13 ..._13_13 def build(self):_13 ..._13_13_13_13_13_13_13
Second, we will import Document
from the langchain.schema module. This will be the return type of the build
method.
_13from langflow import CustomComponent_13from langchain.schema import Document_13_13_13class FlowRunner(CustomComponent):_13 display_name = "Flow Runner"_13 description = "Run other flows using a document as input."_13_13 def build_config(self):_13 ..._13_13 def build(self, flow_name: str, document: Document) -> Document:_13 ..._13_13_13_13_13_13_13
Now, let's add the parameters and the return type to the build
method. The parameters added are:
flow_name
is the name of the flow we want to run.document
is the input document to be passed to that flow.
_14from langflow import CustomComponent_14from langchain.schema import Document_14_14_14class FlowRunner(CustomComponent):_14 display_name = "Flow Runner"_14 description = "Run other flows using a document as input."_14_14 def build_config(self):_14 ..._14_14 def build(self, flow_name: str, document: Document) -> Document:_14 # List the flows_14 flows = self.list_flows()_14_14_14_14_14_14
We can now start writing the build
method. Let's list available flows in "My Collection" using the list_flows
method.
_19from langflow import CustomComponent_19from langchain.schema import Document_19_19_19class FlowRunner(CustomComponent):_19 display_name = "Flow Runner"_19 description = "Run other flows using a document as input."_19_19 def build_config(self):_19 ..._19_19 def build(self, flow_name: str, document: Document) -> Document:_19 # List the flows_19 flows = self.list_flows()_19 # Get the flow that matches the selected name_19 # You can also get the flow by id_19 # using self.get_flow(flow_id=flow_id)_19 tweaks = {}_19 flow = self.get_flow(flow_name=flow_name, tweaks=tweaks)_19
And retrieve a flow that matches the selected name (we'll make a dropdown input field for the user to choose among flow names).
From version 0.4.0, names are unique, which was not the case in previous versions. This might lead to unexpected results if using flows with the same name.
_19from langflow import CustomComponent_19from langchain.schema import Document_19_19_19class FlowRunner(CustomComponent):_19 display_name = "Flow Runner"_19 description = "Run other flows using a document as input."_19_19 def build_config(self):_19 ..._19_19 def build(self, flow_name: str, document: Document) -> Document:_19 # List the flows_19 flows = self.list_flows()_19 # Get the flow that matches the selected name_19 # You can also get the flow by id_19 # using self.get_flow(flow_id=flow_id)_19 tweaks = {}_19 flow = self.get_flow(flow_name=flow_name, tweaks=tweaks)_19
You can load this flow using get_flow
and set a tweaks
dictionary to customize it. Find more about tweaks in our features guidelines.
_26from langflow import CustomComponent_26from langchain.schema import Document_26_26_26class FlowRunner(CustomComponent):_26 display_name = "Flow Runner"_26 description = "Run other flows using a document as input."_26_26 def build_config(self):_26 ..._26_26 def build(self, flow_name: str, document: Document) -> Document:_26 # List the flows_26 flows = self.list_flows()_26 # Get the flow that matches the selected name_26 # You can also get the flow by id_26 # using self.get_flow(flow_id=flow_id)_26 tweaks = {}_26 flow = self.get_flow(flow_name=flow_name, tweaks=tweaks)_26 # Get the page_content from the document
We are using a Document
as input because it is a straightforward way to pass text data in Langflow (specifically because you can connect it to many loaders).
Generally, a flow will take a string or a dictionary as input because that's what LangChain components expect.
In case you are passing a dictionary, you need to build it according to the needs of the flow you are using.
The content of a document can be extracted using the page_content
attribute, which is a string, and passed as an argument to the selected flow.
_32from langflow import CustomComponent_32from langchain.schema import Document_32_32_32class FlowRunner(CustomComponent):_32 display_name = "Flow Runner"_32 description = "Run other flows using a document as input."_32_32 def build_config(self):_32 flows = self.list_flows()_32 flow_names = [f.name for f in flows]_32 return {"flow_name": {"options": flow_names,_32 "display_name": "Flow Name",_32 },_32 "document": {"display_name": "Document"}_32 }_32_32 def build(self, flow_name: str, document: Document) -> Document:_32 # List the flows_32 flows = self.list_flows()
Finally, we can add field customizations through the build_config
method. Here we added the options
key to make the flow_name
field a dropdown menu. Check out the custom component reference for a list of available keys.
Make sure that the field type is str
and options
values are strings.
The typical structure of a Custom Component is composed of display_name
and description
attributes, build
and build_config
methods.
Let's start by defining our component's display_name
and description
.
Second, we will import Document
from the langchain.schema module. This will be the return type of the build
method.
Now, let's add the parameters and the return type to the build
method. The parameters added are:
We can now start writing the build
method. Let's list available flows in "My Collection" using the list_flows
method.
And retrieve a flow that matches the selected name (we'll make a dropdown input field for the user to choose among flow names).
From version 0.4.0, names are unique, which was not the case in previous versions. This might lead to unexpected results if using flows with the same name.
You can load this flow using get_flow
and set a tweaks
dictionary to customize it. Find more about tweaks in our features guidelines.
We are using a Document
as input because it is a straightforward way to pass text data in Langflow (specifically because you can connect it to many loaders).
Generally, a flow will take a string or a dictionary as input because that's what LangChain components expect.
In case you are passing a dictionary, you need to build it according to the needs of the flow you are using.
The content of a document can be extracted using the page_content
attribute, which is a string, and passed as an argument to the selected flow.
Finally, we can add field customizations through the build_config
method. Here we added the options
key to make the flow_name
field a dropdown menu. Check out the custom component reference for a list of available keys.
Make sure that the field type is str
and options
values are strings.
_12from langflow import CustomComponent_12_12_12class MyComponent(CustomComponent):_12 display_name = "Custom Component"_12 description = "This is a custom component"_12_12 def build_config(self):_12 ..._12_12 def build(self):_12 ..._12_12_12_12_12_12_12_12
Done! This is what our script and custom component looks like:
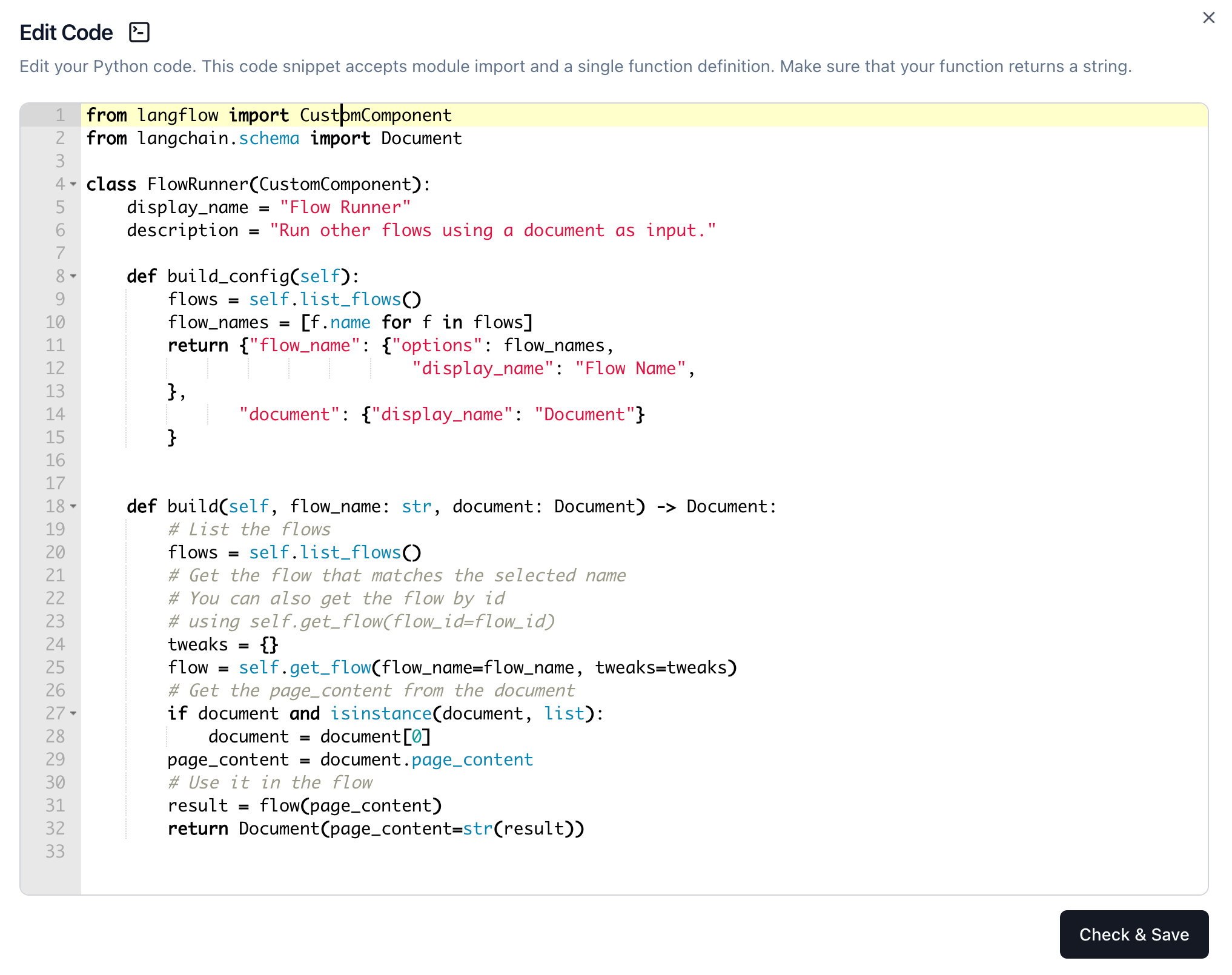
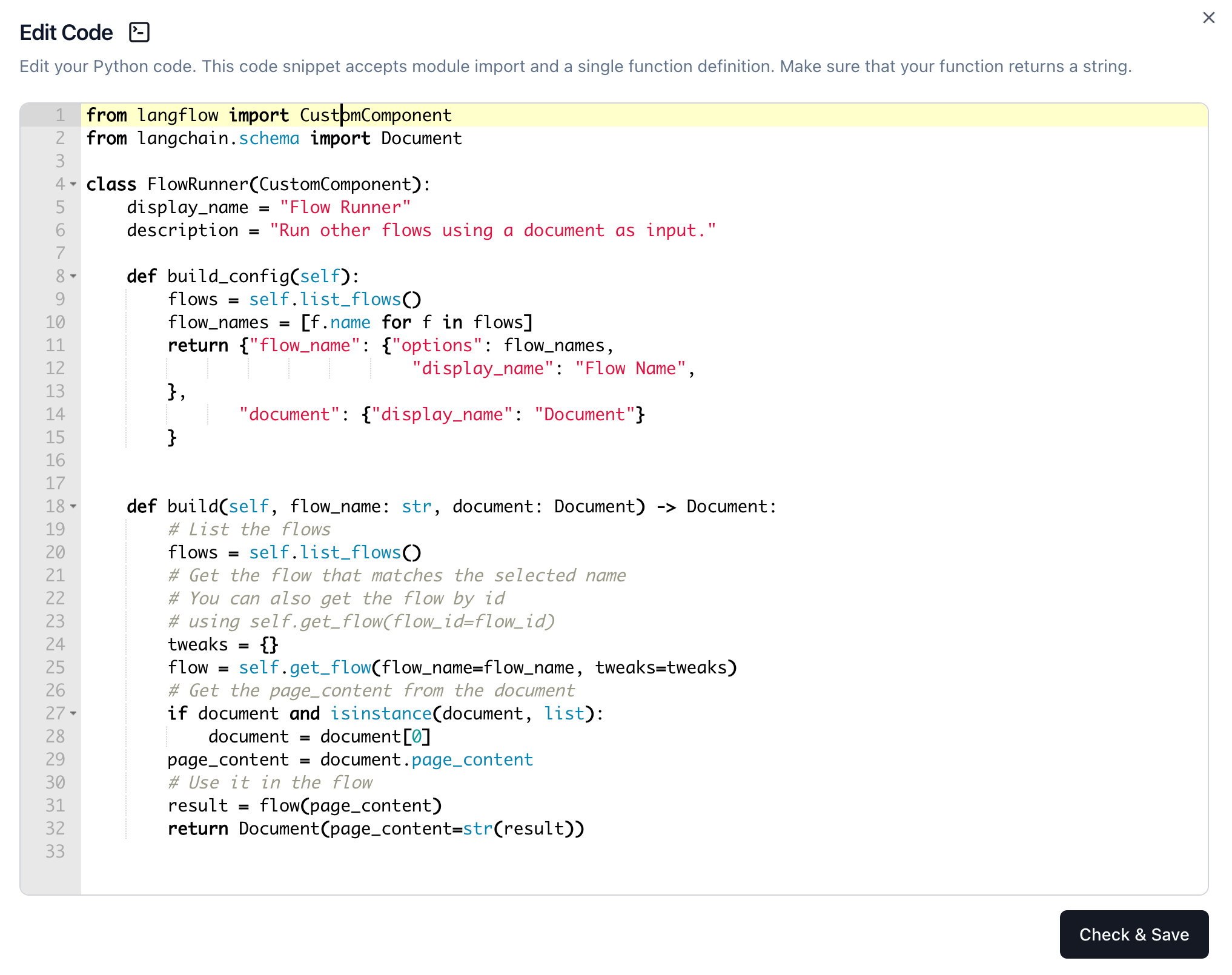
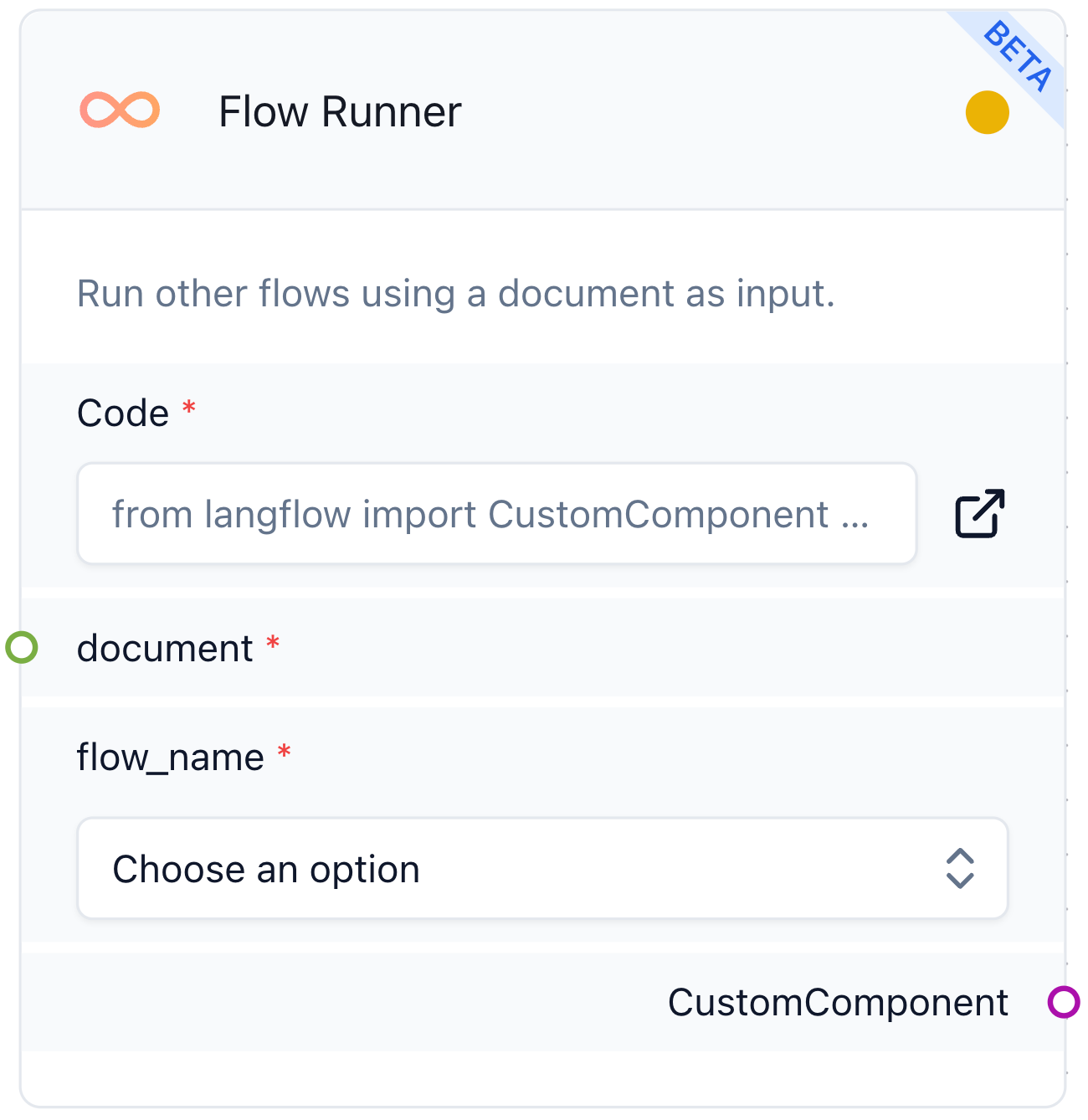
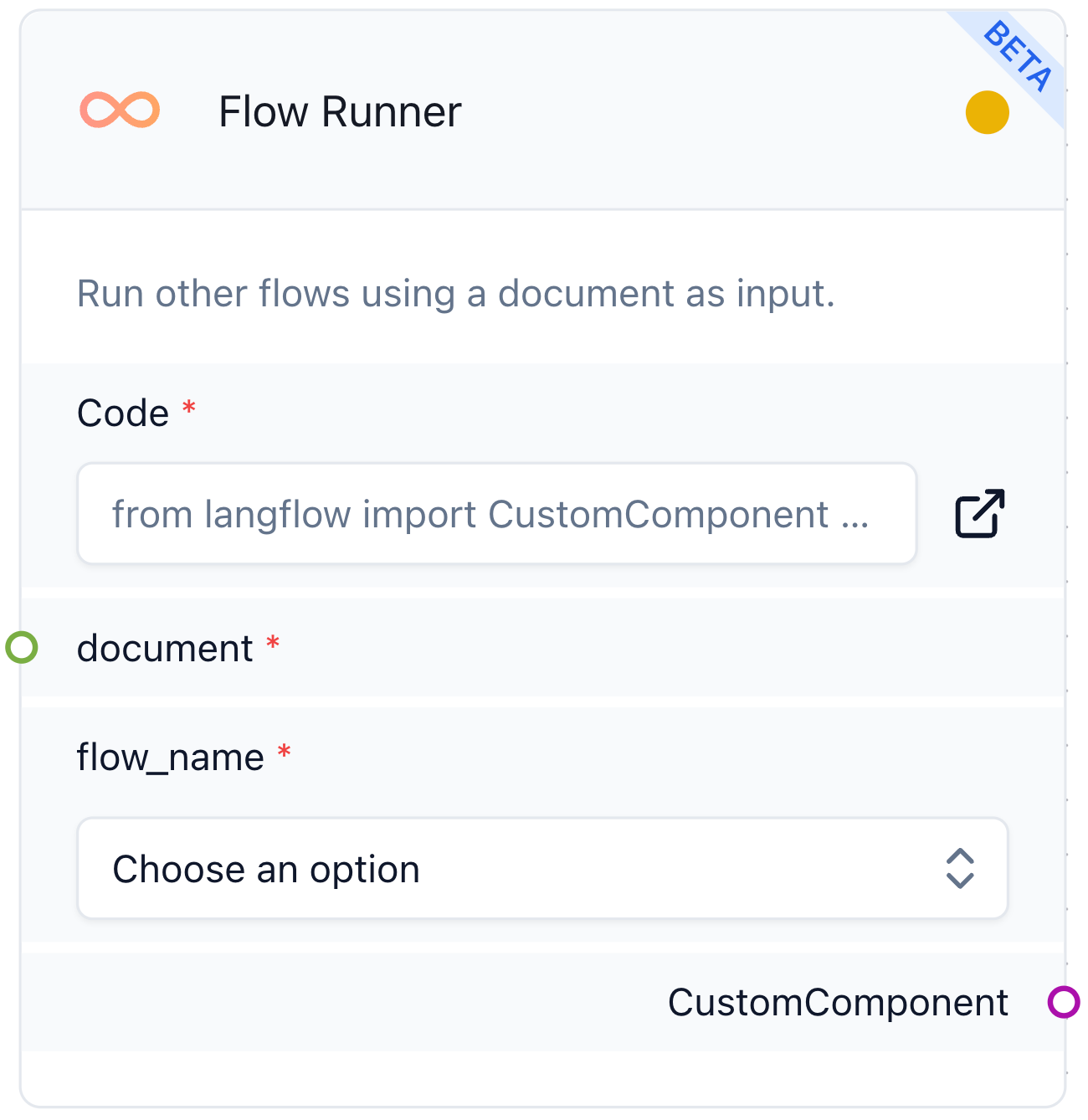